本文共 1161 字,大约阅读时间需要 3 分钟。
大家好!
我是小黄,很高兴又跟大家见面啦 !
拒绝水文,从我做起 !!!!
今天更新的是:
创建时间:2021年3月28日
软件: MindMaster Pro 、Burp Suite Pro 、火狐浏览器
前言
- 在web功能设计中,很多时候我们会要将需要访问的文件定义成变量,从而让前端的功能便的更加灵活。
- 当用户发起一个前端的请求时,便会将请求的这个文件的值(比如文件名称)传递到后台,后台再执行其对应的文件。
- 在这个过程中,如果后台没有对前端传进来的值进行严格的安全考虑,则攻击者可能会通过“…/”这样的手段让后台打开或者执行一些其他的文件。从而导致后台服务器上其他目录的文件结果被遍历出来,形成目录遍历漏洞。
- 看到这里,你可能会觉得目录遍历漏洞和不安全的文件下载,甚至文件包含漏洞有差不多的意思,是的,目录遍历漏洞形成的最主要的原因跟这两者一样,都是在功能设计中将要操作的文件使用变量的方式传递给了后台,而又没有进行严格的安全考虑而造成的,只是出现的位置所展现的现象不一样,因此,这里还是单独拿出来定义一下。
- 需要区分一下的是,如果你通过不带参数的url(比如:http://xxxx/doc)列出了doc文件夹里面所有的文件,这种情况,我们成为敏感信息泄露。而并不归为目录遍历漏洞。(关于敏感信息泄露你你可以在"i can see you ABC"中了解更多)
漏洞分析
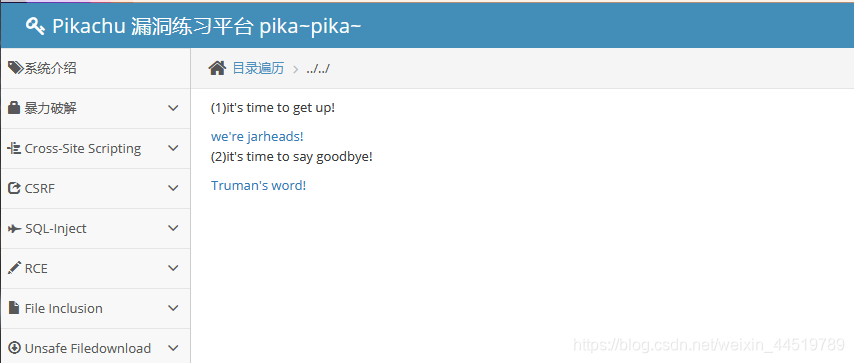
- 根据提示让我们好好读一下这两句话,那好我们就好好读一下
- 我们先打开第一篇文章,来看一下
- 我们顺便记录一下URL:
http://192.168.34.193/pikachu//vul/dir/dir_list.php?title=jarheads.php
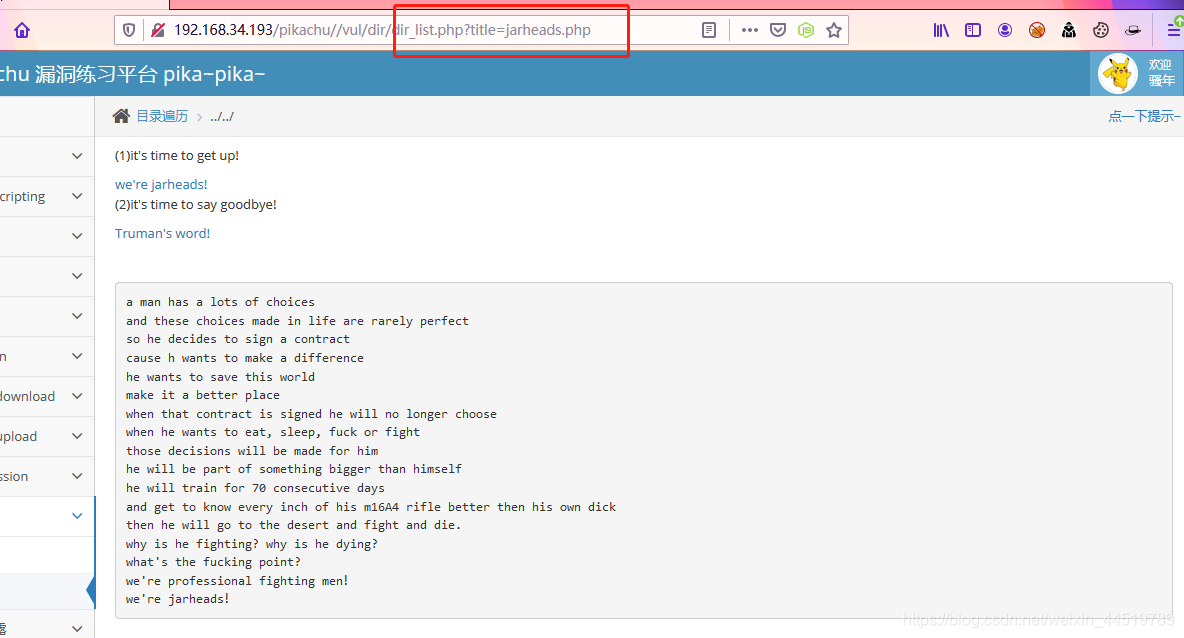
- 我们先打开第二篇文章,来看一下
- 我们顺便记录一下URL:
http://192.168.34.193/pikachu//vul/dir/dir_list.php?title=truman.php
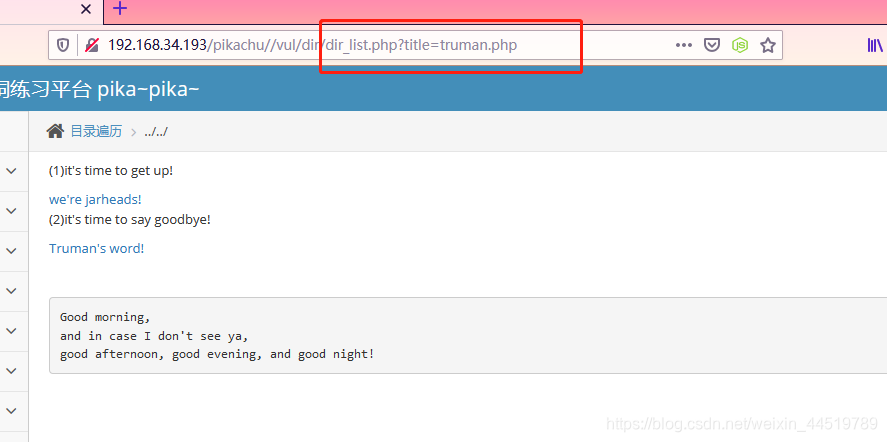
- 通过比对URL发现比对后发现变化的都是
title
- 我们来尝试一下修改
title = ../../
尝试一下
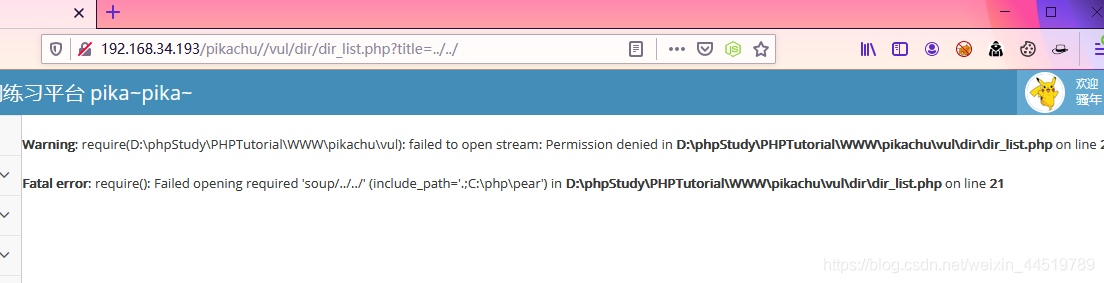
- 这里我们怎么改都报错,我丢
- 我们翻译了一下错误,发现是函数拒绝访问,那我们就去掉他
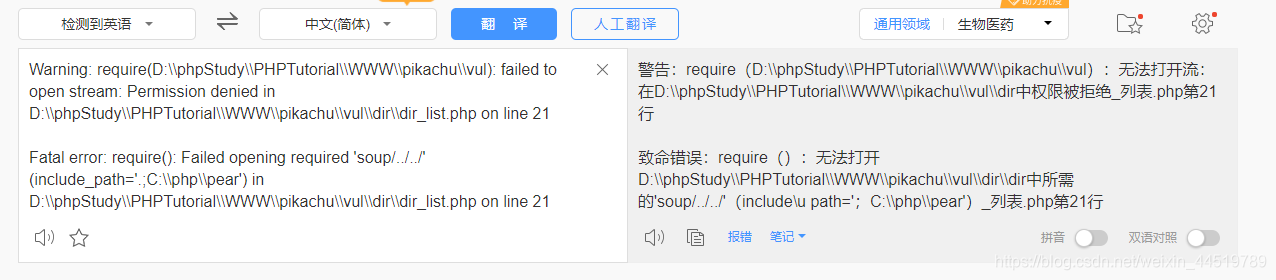
- 重构URL:
192.168.34.193/pikachu//vul/dir/../
- 目录遍历成功
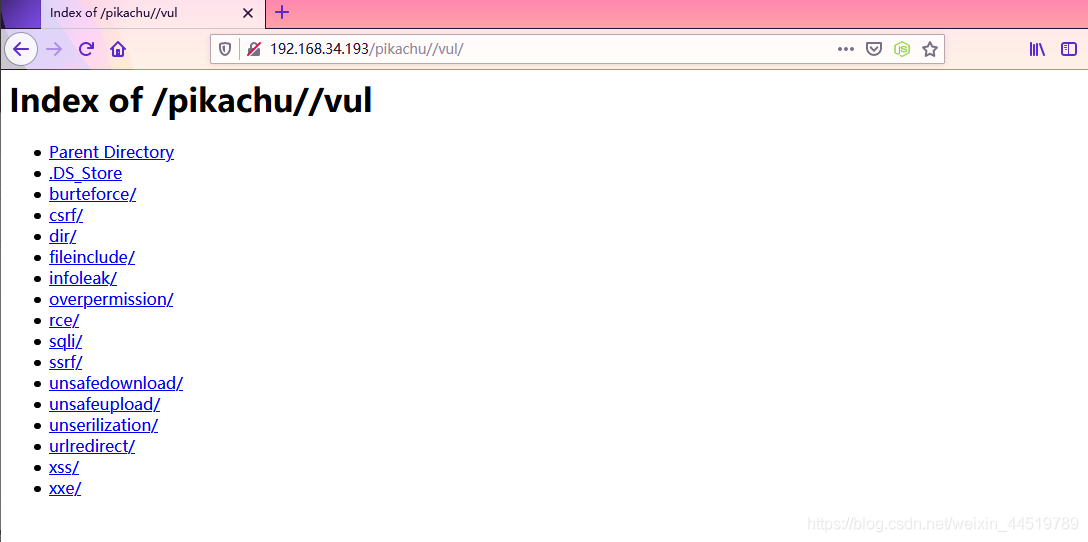
各位路过的朋友,如果觉得可以学到些什么的话,点个赞 再走吧,欢迎各位路过的大佬评论,指正错误,也欢迎有问题的小伙伴评论留言,私信。
每个小伙伴的关注都是本人更新博客的动力!!!
请微信搜索【
在下小黄 】文章更新将在第一时间阅读 !
把握现在 ,展望未来 ,加油 !
由于水平有限 ,写的难免会有些不足之处 ,恳请各位大佬不吝赐教 !
转载地址:http://dvqh.baihongyu.com/